Inheritance in java is a fundamental feature of object-oriented programming (OOP) that allows classes to inherit properties and behavior from other classes. Java, being an object-oriented language, fully supports inheritance, making it an essential aspect of Java programming.
Inheritance enables developers to reuse code, making the code more modular, flexible, and maintainable. It also allows for the creation of more specialized classes by inheriting from existing classes. Java provides a rich set of inheritance features, including single and multiple inheritance, abstract classes, and interfaces.
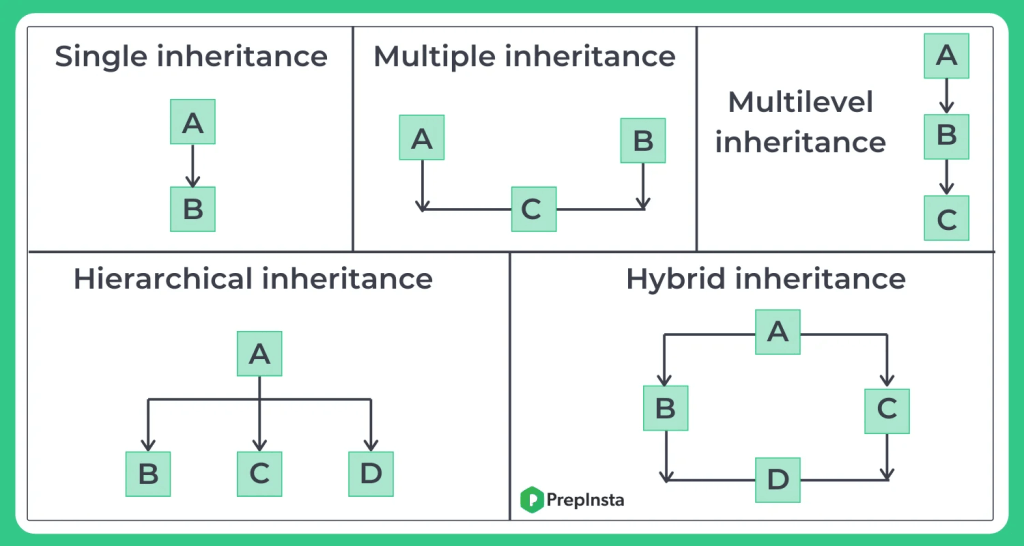
In Java, there are several types of inheritance that can be used to create a hierarchy of classes. These are:
- Single Inheritance: Single inheritance is the simplest type of inheritance, where a subclass inherits from only one superclass. In this case, the subclass has access to all the public and protected methods and fields of the superclass. This is the most commonly used type of inheritance in Java.
Here’s an example of single inheritance in Java:
class Superclass {
public void display() {
System.out.println("This is the superclass.");
}
}
class Subclass extends Superclass {
public void display() {
System.out.println("This is the subclass.");
}
}
In this example, the Subclass inherits the display() method from the Superclass.
- Multilevel Inheritance: Multilevel inheritance is where a subclass inherits from a superclass, and that superclass is itself a subclass of another superclass. This creates a chain of inheritance, where the subclass has access to the methods and fields of all the superclasses in the chain.
Here’s an example of multilevel inheritance in Java:
class Superclass {
public void display() {
System.out.println("This is the superclass.");
}
}
class Subclass1 extends Superclass {
// Subclass1 methods and fields
}
class Subclass2 extends Subclass1 {
public void display() {
System.out.println("This is the subclass2.");
}
}
In this example, Subclass2 inherits from Subclass1, which in turn inherits from Superclass.
- Hierarchical Inheritance: Hierarchical inheritance is where multiple subclasses inherit from a single superclass. This creates a hierarchy of classes, where each subclass has access to the methods and fields of the superclass.
Here’s an example of hierarchical inheritance in Java:
class Superclass {
public void display() {
System.out.println("This is the superclass.");
}
}
class Subclass1 extends Superclass {
// Subclass1 methods and fields
}
class Subclass2 extends Superclass {
// Subclass2 methods and fields
}
In this example, both Subclass1 and Subclass2 inherit from Superclass.
- Multiple Inheritance: Multiple inheritance is where a subclass inherits from multiple superclasses. In Java, multiple inheritance is not directly supported, but it can be achieved using interfaces. An interface is a collection of abstract methods that a class can implement to provide its own implementation.
Here’s an example of multiple inheritance using interfaces in Java:
interface Interface1 {
public void display1();
}
interface Interface2 {
public void display2();
}
class Subclass implements Interface1, Interface2 {
public void display1() {
System.out.println("This is the first interface.");
}
public void display2() {
System.out.println("This is the second interface.");
}
}
In this example, Subclass implements both Interface1 and Interface2, which allows it to access the methods of both interfaces.
Conclusion: In Java, inheritance is an essential concept that allows classes to inherit properties and methods from other classes. There are several types of inheritance that can be used to create a hierarchy of classes, including single inheritance, multilevel inheritance, hierarchical inheritance, and multiple inheritance using interfaces. Each type of inheritance has its own advantages and disadvantages, and understanding the different types is essential for creating effective class hierarchies.