What is Method Overloading in Java?
Method overloading is a powerful feature of the Java programming language that allows developers to define multiple methods with the same name but different parameters within a class. It provides a convenient way to create numerous variations of a method, each tailored to handle different input types or numbers of arguments. Method overloading enhances code readability, reusability, and flexibility by enabling developers to write concise and intuitive code.
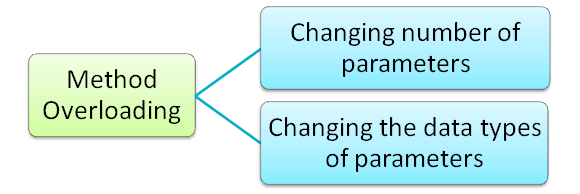
When two or more methods within a class have the same name but different parameters, they are said to be overloaded. The compiler distinguishes between these methods based on the number of parameters, types, and order. During compilation, the Java compiler matches the method call with the appropriate method definition based on the arguments passed. This process is known as static or compile-time polymorphism, as the decision of which method to invoke is made at compile-time.
How do you achieve method overloading?
To illustrate the concept of method overloading, consider the following example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In this example, the Calculator class defines two add methods: one that takes two integer parameters and another that takes two double parameters. These methods have the same name but different parameter types. When calling the add method, the compiler determines the appropriate method to invoke based on the argument types:
Calculator calculator = new Calculator();
int sum1 = calculator.add(2, 3); // Invokes the add(int, int) method
double sum2 = calculator.add(2.5, 3.7); // Invokes the add(double, double) method
The arguments are integers in the first method call, so the add(int, int)
method is invoked. In the second method call, the arguments are doubles, so the add(double, double)
method is invoked. Method overloading allows developers to use the same method name (add
) to perform addition with different data types, making the code more concise and readable.
Method overloading is not limited to just the number or types of parameters. It can also differ based on the order of the parameters or a combination of these factors. Let’s consider another example to demonstrate this:
public class StringUtils {
public void concatenate(String str1, String str2) {
System.out.println(str1 + str2);
}
public void concatenate(String str1, String str2, String str3) {
System.out.println(str1 + str2 + str3);
}
public void concatenate(String str1, int number) {
System.out.println(str1 + number);
}
}
In this example, the StringUtils
class defines three concatenate
methods that differ in both the number and types of parameters. The first concatenate
the method takes two strings, the second takes three strings, and the third takes a string and an integer. The order and type of parameters help the compiler distinguish between these methods.
StringUtils utils = new StringUtils();
utils.concatenate("Hello, ", "world!"); // Invokes the concatenate(String, String) method
utils.concatenate("Hello, ", "world!", " Welcome!"); // Invokes the concatenate(String, String, String) method
utils.concatenate("The number is: ", 42); // Invokes the concatenate(String, int) method
In the above code, the appropriate concatenate
method is invoked based on the number and types of arguments provided. The method overloading feature enables developers to create methods that can handle different scenarios while maintaining a consistent method name and avoiding the need for multiple method names for similar operations.
Method overloading is widely used in Java libraries and frameworks. For example, the println
method of the PrintStream
class in the java.io
package is overloaded to handle various data types. It provides different versions of the method to print different types of values, such as integers, booleans, doubles, and objects.
There are a few considerations to keep in mind when using method overloading. First, the return type of the method does not play a role in overloading. Two methods with the same name and parameters but different return types will result in a compilation error. Second, the methods being overloaded must be in the same class. Overloading does not work across different classes or subclasses. Lastly, the compiler determines the most specific matching method for a given method call. If there is an exact match, that method is invoked. Otherwise, the compiler looks for the closest possible match by promoting the arguments if necessary.
Conclusion
In conclusion, method overloading in Java is a powerful feature that allows developers to create multiple methods with the same name but different parameters. It enhances code readability and reusability by providing a concise and intuitive way to handle different scenarios. By overloading methods, developers can write cleaner code that is easier to understand and maintain. Understanding method overloading is essential for any Java developer to leverage the full potential of the language and write efficient and flexible code. Click here to check the official documentation