PHP beginner tutorials in 2020 | PHP beginner tutorials
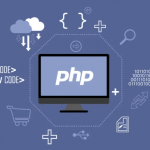
What is PHP?
PHP beginner tutorials | PHP stands for Hypertext Preprocessor. PHP is a most powerful and widely-used open-source server-side scripting language. PHP scripting language is used to write dynamically generated web pages. PHP scripts are executed on the web servers and the final result is sent to the browser as plain content with the help of HTML tags.
for Hypertext Preprocessor (PHP) is an open-source, interpreted, and object-oriented scripting language that means it is executed at the server-side. It is used to develop web applications these applications are executed at the server-side and generates a dynamic web page.
What is PHP
- PHP is a server-side scripting language.
- It is an interpreted language that means there is no need for compilation.
- Object-oriented language.
- It is an open-source scripting language.
- It is simple and easy to learn the language.
PHP can be easily embedded within a normal HTML web page. That means inside your HTML documents you can write PHP statements like this:
<!DOCTYPE HTML> <html> <head> <title>My First PHP Application</title> </head> <body> <?php // Program to display simple greeting message echo 'Hello World!'; ?> </body> </html>
PHP Features
PHP has many features some of them are listed below:
- Performance: PHP scripts are executing much faster than those scripts written in other languages such as JSP & ASP.
- Open Source: PHP is open-source software available on the internet, you can develop your website with all the version of PHP according to your requirement without paying any cost.
- Platform Independent: PHP are available for WINDOWS, MAC, LINUX & UNIX OS.
- Compatibility: It is compatible with almost all the local servers available and used today like Apache, IIS etc.
- Embedded: We can embed PHP code easily within HTML tags and script.
What You Can Do with PHP?
There are unlimited things that you can do with PHP.
- You can generate dynamic web pages and files.
- Can create, open, read, write, and close files on the webserver.
- Collect data using a web form such as user information, email ids, credit card information, and many more.
- I can send emails to the users of your website.
- Cookies can send and receive to track the visitor information of your website.
- Information’s can be the store, delete, and modify in the database.
- Restrict unauthorized access to your website.
- Data can be encrypted for safe data transmission over the internet.
Learn how you can encrypt data transmission using SSL
PHP tags always start with <?php and end with ?>
Embedding PHP Scripts
There are four different ways that you can embed your PHP code.
- You can embed PHP code into a standard HTML page. To do it you have to put PHP code between <? and ?> tags.
Example:
<html> <head> <title>Simple PHP Code</title> </head> <body> <? echo "Hello World, Stay Safe"; ?> </body> </html>
Above mentioned embedding style is the most common way to embed your PHP code.
- Another most popular way to embed PHP code is within <?php and ?> tags:
<?php echo "Hello World, Stay safe"; ?>
This style is always available and is recommended when your PHP code needs to be portable to many different systems.
- Embedding PHP within<script> tags is another style that is always available:
<script language="php" > echo "Hello World";</script>
- One final style, in which the code is between <% and %> tags, is disabled by default:
<% echo "Hello World"; %>
Creating a variable in PHP
Variables are the name or some symbols that are used to store values such as numeric values, memory addresses, characters, etc.
Most required Rules for PHP variables:
- Variables start with $ sign and followed by the name of your variable.
- A variable must start with an underscore or letter.
- The variable name cannot start with numbers in PHP.
- It can only contain alphanumeric and underscore (A-z, 0-9, and _).
- Variable names are case sensitive.
<?php $sampleVariable = "Hello, this is example variable"; echo "message in variable: $sampleVariable "; ?>
Decision-making statements:
PHP supports three types of decision-making statements, they are
- if..else statement
- else if statement
- switch statement
Example of if..else statement
<?php $num=156; if($num%2==0){ echo "$num is even number"; }else{ echo "$num is odd number"; } ?>
else if statement
<?php $x = 10; if ( $x < 0 ) { echo "x is negative."; } elseif ( $x == 0 ) { echo "x is equal to 0"; } else { echo "x is positive"; } echo " value of x = " . $x; ?> //Output "x is positive" and "value of x = 10".
switch statement
<?php $myfavcolor = "black"; switch ($myfavcolor) { case "red": echo "Your favorite color is red!"; break; case "blue": echo "Your favorite color is blue!"; break; case "green": echo "Your favorite color is green!"; break; case "black": echo "Your favorite color is black!"; break; default: echo "Your favorite color is neither red, blue, nor green!"; } ?>
Functions in PHP
- In PHP function name always starts with a small letter, underscore (_).
- The function name cannot start with numbers.
- Never use the predefined constant name, for example, PHP_OS.
- Never use reserved keywords like, if, else, etc.
- Case insensitive.
Syntax:
function fun_name(){ Blocks of statements; }
Example:
function displayMsg(){ echo "Hello world, stay safe."; }
Now to display the above message we need to call the function in order to print the message.
Syntax calling Function:
Function_name();
Above Program to call the method.
function displayMsg(){ echo "Hello world, stay safe."; } displayMsg();