Table of Contents
What is functions in C programming?
C Programming language also support functions like other programming languages. Functions is the set of statements that takes input, perform some operation and produces output.
Syntax,
return_type fun-name(set of inputs)
Why function?
Pointers in C programming Language
https://studywholenight.com/pointers-in-c-programming-language/
Function provides you the flexibility of managing code. I have listed major reasons why we must use functions in c or on any other programming languages.
- Reusability: You can reuse same code over and over without writing same codes again and again.
- Abstraction: If you are using the function in your program, then you don’t have to worry about how it works inside. for example scanf() function.
- Function can divide a large program code into smaller pieces.
- Function is declared to tell a computer about it’s existence.
- A function is called in order to be used.
- Function is defined to get some task done in easy way.
Types of functions in c programming
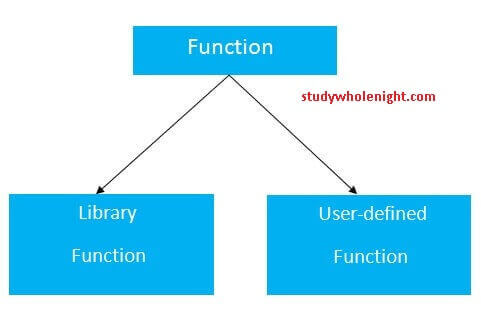
a) Library function is the inbuilt functions in C programming language. built-in functions are defined inside header files. For example if we want to use printf() function then we need to include #include<stdio.h> in our program.
b) User Defined Function: User defined functions are created by programmer. We can create functions as per our need.
Basic Structure of function in C programming Language.
#include<stdio.h>
int myFunction()
{
.............
.............
}
int main()
{
............
............
myFunction();
}
Program to add two numbers in C programming using function
- Creating Allure Report With Cucumber – Easy Steps
- How do I make an HTTP request in JavaScript – Best Approach?
- Java Objects: A Comprehensive Guide from Basics to Intermediate Concepts
- Exploring Method Overloading in Java: Powerful Programming Technique
- Mastering Java Enum: A Comprehensive Guide for Developers
#include<stdio.h>
int sum(int a, int b);
int main()
{
int a, b, c;
a= 5;
b= 12;
c = sum(a+b);
printf("The sum is: %d",c);
return 0;
}
int sum(int a, int b)
{
return a+b;
}